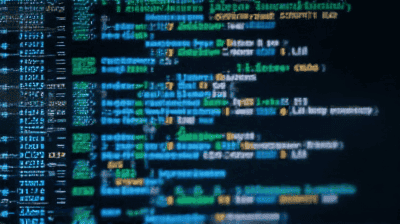
In the ever-evolving landscape of computer science, trends come and go, technologies become obsolete while new paradigms rise to prominence. One such paradigm that has recently experienced a resurgence is functional programming. Originally developed in the 1950s, functional programming is gaining renewed attention in the tech world for its powerful abstractions, elegance, and ability to manage complexity in software design.
Understanding Functional Programming
What is Functional Programming?
At its core, functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. This approach emphasizes the application of functions, often leading to more predictable and reliable code. Key concepts in functional programming include:
- First-Class Functions: Functions are treated as first-class citizens, meaning they can be assigned to variables, passed as arguments, and returned from other functions.
- Immutability: Once a value is created, it cannot be changed. This eliminates side effects and makes reasoning about code easier.
- Pure Functions: A function's output is determined solely by its input values, without observable side effects.
- Higher-Order Functions: Functions that can take other functions as arguments or return them as results, allowing for powerful abstractions.
The History of Functional Programming
Functional programming has its roots in mathematical logic and the lambda calculus developed by Alonzo Church in the 1930s. While languages like Lisp, introduced in 1958, brought functional programming to the forefront, the paradigm faced competition from imperative and object-oriented programming (OOP) models throughout the latter half of the 20th century.
However, with the advancements in multi-core processing, distributed systems, and the need for more robust software architectures, the limitations of imperative languages have become more apparent. Consequently, the elegance and power of functional programming concepts have resurfaced, leading to a renaissance in the tech community.
The Rise of Functional Programming
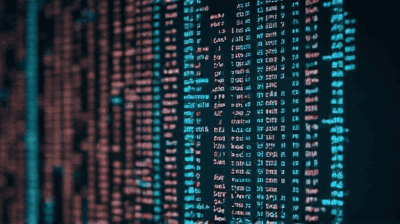
Addressing Modern Software Challenges
As software systems have grown in complexity, developers have faced numerous challenges that traditional programming paradigms struggle to address. Key issues include:
Concurrency and Parallelism: The increasing reliance on multi-core processors has highlighted the difficulties of managing shared state in imperative programming. Functional programming's emphasis on immutability and pure functions simplifies concurrent programming and reduces the risk of race conditions.
Maintaining Code Quality: As software projects scale, maintaining code quality becomes increasingly difficult. Functional programming encourages writing clean, elegant code through its focus on functions, facilitating easier testing and debugging.
Complexity Management: Functional languages offer powerful abstractions for managing complexity, such as algebraic data types and higher-order functions. These abstractions can help developers build more robust applications while reducing the cognitive overhead associated with imperative programming styles.
Language Support for Functional Programming
Numerous programming languages support functional programming techniques, either as their primary paradigm or as a blend with other paradigms. Some notable languages include:
- Haskell: A purely functional programming language known for its strong static typing and lazy evaluation, making it a favorite among functional programming purists.
- Scala: A hybrid language that combines OOP and functional programming, popular for its concise syntax and powerful abstractions.
- Elixir: A functional, concurrent language built on the Erlang VM, specializing in distributed and fault-tolerant systems.
- JavaScript: Originally an imperative language, JavaScript has adopted many functional features, making it popular for web development.
The versatility of these languages has contributed to the growing acceptance of functional programming concepts across various domains.
Key Concepts in Functional Programming
Immutability
Immutability is a cornerstone of functional programming. When a data structure is immutable, any attempt to modify it results in the creation of a new instance rather than changing the original. This practice leads to several advantages:
- Predictability: Because immutable data cannot change, developers can reason about their code more easily. This predictability is crucial in concurrent applications where multiple threads may access data.
- Easier Debugging: Since the state of data does not change, the need for complex debugging techniques associated with mutable state is minimized.
- Referential Transparency: Function outputs depend solely on their inputs, making functions easier to test and reason about.
First-Class and Higher-Order Functions
Functional programming heavily emphasizes the use of first-class and higher-order functions. These concepts allow developers to build powerful abstractions and facilitate code reuse:
- First-Class Functions: In languages that treat functions as first-class citizens, developers can store functions in variables, pass them as arguments, and return them from other functions. This leads to greater flexibility in designing software.
- Higher-Order Functions: Functions that take other functions as arguments or return functions as results enable developers to create more abstract and reusable components. Examples of higher-order functions include
map
,filter
, andreduce
, which allow for concise and expressive operations on collections.
Pure Functions and Side Effects
A pure function is defined as a function that, given the same input values, will always return the same output and has no side effects. Side effects refer to any interactions with the outside world, such as modifying global variables, I/O operations, or database transactions.
The importance of pure functions lies in their predictability and testability. Since their output is entirely dependent on the input, pure functions can be easily tested in isolation, promoting modularity and reusability.
Benefits of Functional Programming
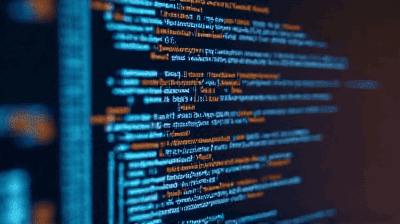
As the tech industry increasingly embraces functional programming, several key benefits are becoming apparent:
1. Improved Maintainability
Functional programming's emphasis on immutability, pure functions, and modular design contributes to improved maintainability. As a codebase evolves, the likelihood of unintended side effects is reduced, making it easier to modify and extend software without introducing new bugs.
2. Enhanced Testability
The use of pure functions and the absence of side effects make functional programs inherently easier to test. Developers can write unit tests with confidence, knowing that a function's output is consistent and predictable, leading to more reliable software.
3. Concurrency and Parallelism
As previously mentioned, functional programming simplifies concurrency through its avoidance of mutable state. This design choice makes it easier to develop applications that leverage multi-core processors, ultimately leading to better utilization of modern hardware.
4. Concise and Expressive Code
Functional programming allows for writing code that is both concise and expressive. Higher-order functions and powerful abstractions enable developers to express complex operations in fewer lines of code, enhancing readability and maintainability.
Real-World Applications of Functional Programming
Functional programming is making its mark across various domains and industries. Here are some notable examples:
Data Processing and Analysis
Functional programming languages like Scala and Python (with libraries like Pandas) are widely used in data processing and analytics. Their ability to handle large datasets through functions enables efficient and parallel processing.
Web Development
JavaScript's functional programming capabilities have transformed web development. Libraries and frameworks like React embrace functional programming principles, allowing for the development of dynamic user interfaces with manageable state.
Distributed Systems
Functional languages like Elixir and Erlang are well-suited for building distributed systems, thanks to their emphasis on concurrency and fault tolerance. Companies like WhatsApp use Erlang to handle millions of concurrent connections reliably.
Financial Services
The financial industry is increasingly adopting functional programming due to its ability to model complex financial systems and support high-performance computations. Languages like Haskell and F# are favored for their strong type systems and mathematical expressiveness.
Challenges of Adopting Functional Programming
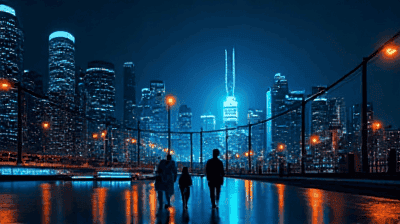
Despite its benefits, adopting functional programming may come with challenges. Some potential hurdles include:
Learning Curve
For developers accustomed to imperative programming, transitioning to a functional style can be challenging. The shift in mindset, coupled with new concepts such as immutability and higher-order functions, may require time and practice.
Performance Considerations
While functional programming can simplify code and enhance maintainability, it may introduce performance overhead in certain scenarios. For example, immutability can result in additional memory allocations, which can lead to performance challenges in performance-critical applications.
Tooling and Ecosystem Maturity
While many functional programming languages have matured significantly, others may lack the extensive libraries, frameworks, and tooling that popular imperative languages offer. Developers may need to invest additional effort in building the necessary infrastructure for functional programming applications.
The Future of Functional Programming
The functional programming renaissance shows no signs of slowing down. As the tech industry continues to grapple with increasing complexity, concurrency, and the need for maintainable code, the principles of functional programming will remain relevant.
Educating the Next Generation of Developers
As formal education evolves, incorporating functional programming concepts into curricula will be crucial. Familiarizing students with different programming paradigms will diversify their skill sets and prepare them for the demands of modern software development.
Hybrid Approaches
Many modern languages embrace a hybrid approach, combining functional programming with imperative or object-oriented paradigms. Developers can leverage the strengths of each paradigm, creating applications that are both performant and maintainable.
Advancements in Language Design and Performance
As research in language design and optimization techniques continues, we can expect functional programming languages to become more performant and accommodating for broader applications. Enhanced type systems, better compiler optimizations, and advanced tooling will facilitate wider adoption.
Conclusion
The revival of functional programming in the tech industry is a testament to the enduring power of its concepts. As developers confront the realities of complexity, concurrency, and the evolving needs of software design, the elegant abstractions and principles of functional programming offer valuable solutions.
By integrating functional programming techniques into their skill set, developers can create more predictable, maintainable, and efficient code. As we continue to explore and innovate in the realm of software development, the functional programming paradigm remains a vital part of the ongoing journey toward building better software systems.